- Suppose we have a text file votes.txt of integer data containing all the votes cast in an election. This election happened to have three candidates and the values in the integer file are 1, 2, or 3, each corresponding to one of the three candidates.
Code Sample 16-2:
FileInput inFile = new FileInput("votes.txt");
int vote, total = 0, loop;
// sized to 4 boxes, initialized to 0's
int[] data = new int[4];
vote = inFile.readInt();
while (inFile.hasMoreTokens()){
data[vote]++;
total++;
vote = inFile.readInt();
}
System.out.println("Total # of votes = " + total);
for (loop = 1; loop <= 3; loop++){
System.out.println("Votes for #" + loop +
" = " + data[loop]);
}
- The array
data
consists of four cells, each holding an integer value. The first cell, data[0]
, is allocated but not used in this problem. After processing the entire file, the variable data[n]
contains the number of votes for candidate n. We could have stored the information for candidate 1 in position 0, candidate 2 in position 1, and so forth, but the code is easier to follow if we can use a direct correspondence.
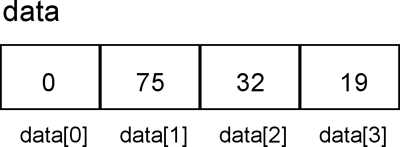
- The value of vote is used to increment the appropriate cell of the array by +1.
A second example counts the occurrence of each alphabet letter in a text file.
Code Sample 16-3:
FileInput inFile = new FileInput("sample.txt");
int[] letters = new int[26]; // use positions 0..25
// to count letters
int total = 0;
char ch;
while (inFile.hasMoreLines()){
String line = inFile.readLine().toLowerCase();
for(int index = 0; index < line.length(); index++){
ch = line.charAt(index);
// line.charAt is from chn.util. It
//extracts the entry.
if ('a' <= ch && ch <= 'z') { // if letter
letters[ch - ‘a’]++;
total++;
}
}
}
System.out.println("Count letters");
System.out.println();
ch = 'a';
for (int loop = 0; loop < 26; loop++){
System.out.println(ch + " : " + letters[loop]);
ch++;
}
System.out.println();
System.out.println("Total letters = " + total);
-
Each line in the text file is read in and then each character in the line is copied into ch
. If ch
is an uppercase letter, it is converted to its lowercase counterpart.
- If the character is a letter, the ASCII value of the letter is adjusted to fit the range from 0-25. For example, if
ch == 'b'
, the program calculates ‘b’ - ‘a’ = 1
. Then the appropriate cell of the array is incremented by one.